|
| 1 | +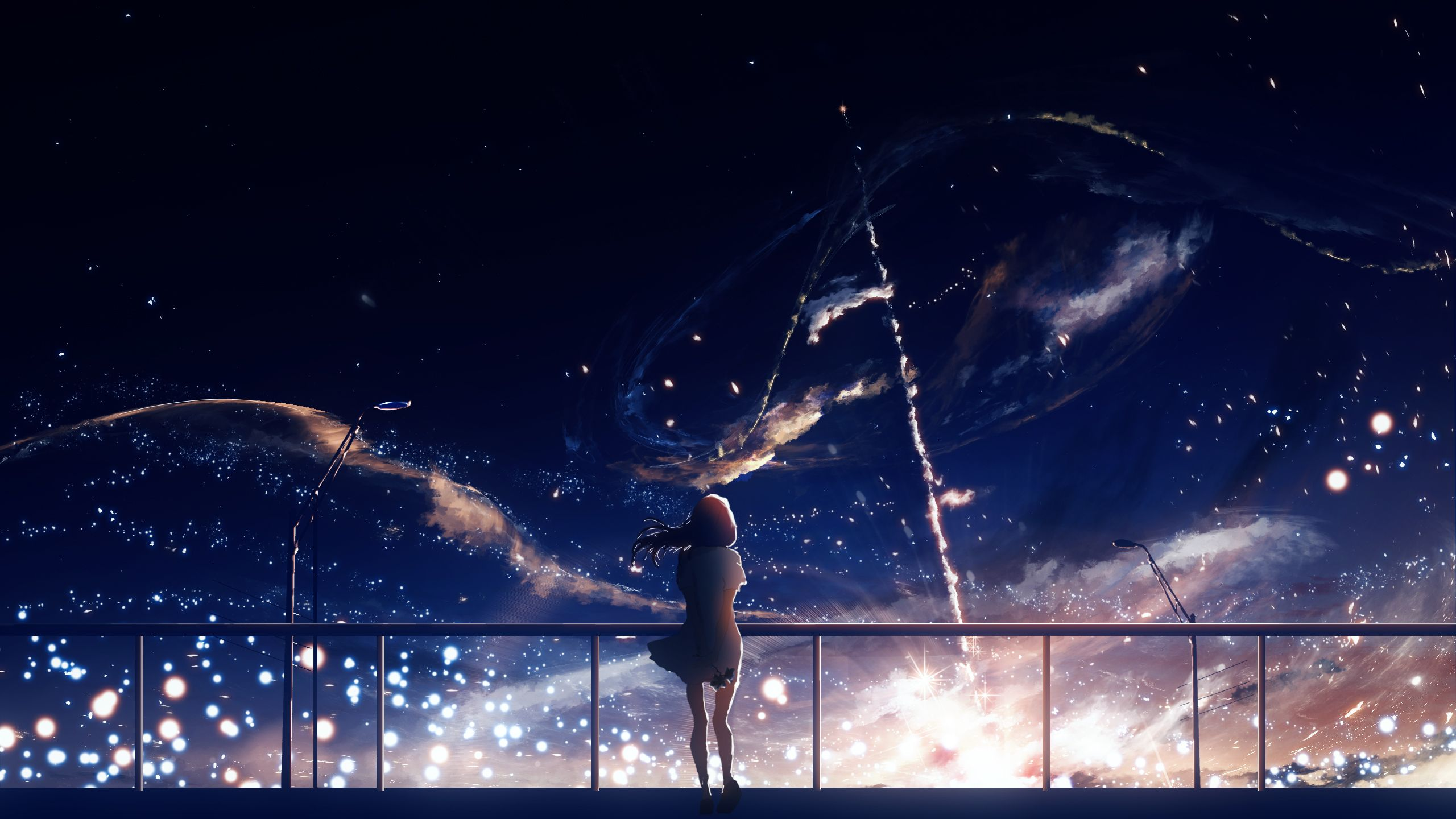 |
| 2 | +>仰望星空的人,不应该被嘲笑 |
| 3 | + |
| 4 | +## 题目描述 |
| 5 | + |
| 6 | +给定一个二叉树,检查它是否是镜像对称的。 |
| 7 | + |
| 8 | + |
| 9 | + |
| 10 | +例如,二叉树 [1,2,2,3,4,4,3] 是对称的。 |
| 11 | + |
| 12 | +```javascript |
| 13 | + 1 |
| 14 | + / \ |
| 15 | + 2 2 |
| 16 | + / \ / \ |
| 17 | +3 4 4 3 |
| 18 | +``` |
| 19 | + |
| 20 | + |
| 21 | + |
| 22 | +但是下面这个 [1,2,2,null,3,null,3] 则不是镜像对称的: |
| 23 | + |
| 24 | +```javascript |
| 25 | + 1 |
| 26 | + / \ |
| 27 | + 2 2 |
| 28 | + \ \ |
| 29 | + 3 3 |
| 30 | + |
| 31 | +``` |
| 32 | + |
| 33 | +进阶: |
| 34 | + |
| 35 | +你可以运用递归和迭代两种方法解决这个问题吗? |
| 36 | + |
| 37 | +来源:力扣(LeetCode) |
| 38 | +链接:https://leetcode-cn.com/problems/symmetric-tree |
| 39 | +著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。 |
| 40 | + |
| 41 | + |
| 42 | + |
| 43 | +## 解题思路 |
| 44 | +`dfs`,逐层进行比较,即自顶向底找,注意判断几个条件: |
| 45 | + |
| 46 | +- 如果左右节点都为空,可以 |
| 47 | +- 如果左右节点一个为空,不可以 |
| 48 | +- 如果左右节点值不相等,不可以 |
| 49 | +- 最后递归左右子树镜像 |
| 50 | + |
| 51 | +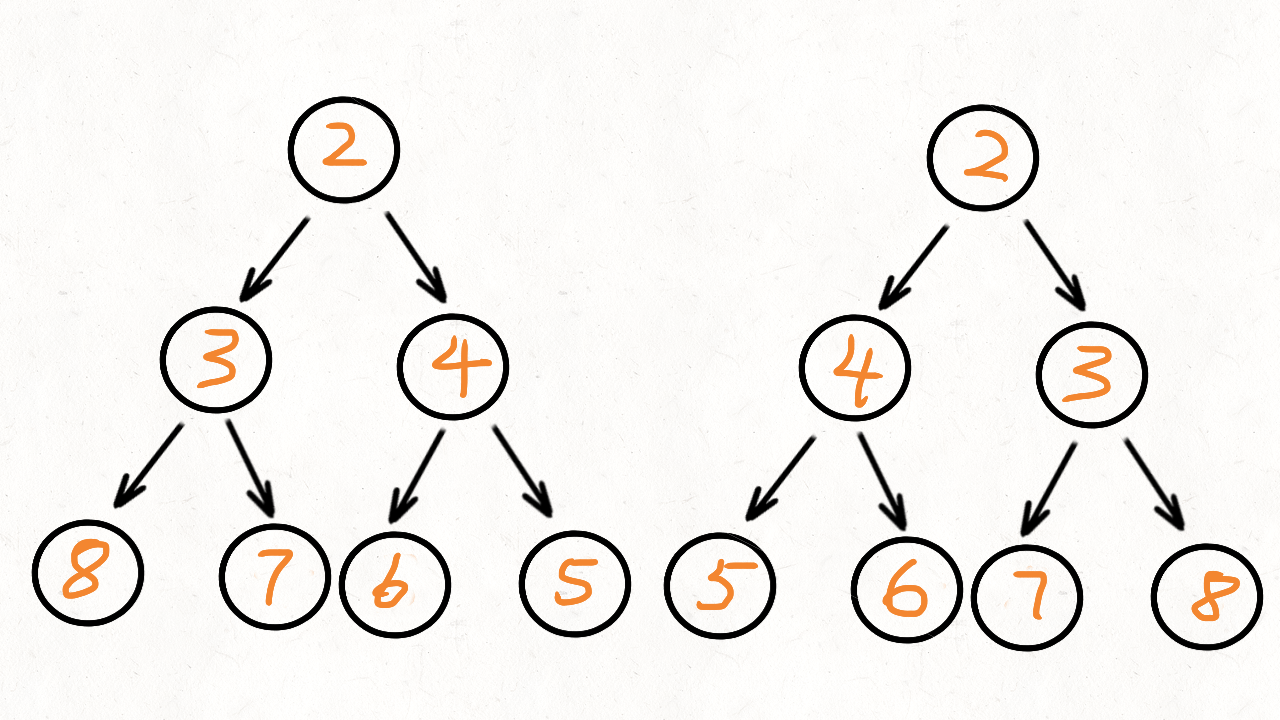 |
| 52 | +参考 <a href="https://leetcode-cn.com/problems/symmetric-tree/solution/dong-hua-yan-shi-101-dui-cheng-er-cha-shu-by-user7/">王尼玛</a> 大佬图解 |
| 53 | + |
| 54 | +```javascript |
| 55 | +/** |
| 56 | + * Definition for a binary tree node. |
| 57 | + * function TreeNode(val) { |
| 58 | + * this.val = val; |
| 59 | + * this.left = this.right = null; |
| 60 | + * } |
| 61 | + */ |
| 62 | +/** |
| 63 | + * @param {TreeNode} root |
| 64 | + * @return {boolean} |
| 65 | + */ |
| 66 | +var isSymmetric = function (root) { |
| 67 | + if (!root) return true; |
| 68 | + let dfs = (left, right) => { |
| 69 | + // 如果左右节点都为空,可以 |
| 70 | + if (left == null && right == null) return true; |
| 71 | + // 如果左右节点一个为空,不可以 |
| 72 | + if(left == null || right == null) return false; |
| 73 | + // 如果左右节点值不相等,不可以 |
| 74 | + if (left.val !== right.val) return false; |
| 75 | + // 递归左右子树镜像 |
| 76 | + return dfs(left.left, right.right) && dfs(left.right, right.left); |
| 77 | + } |
| 78 | + return dfs(root.left, root.right); |
| 79 | +}; |
| 80 | +``` |
| 81 | +**解法二** |
| 82 | + |
| 83 | +通过队列逐步一层一层来比较,只要出现不对称的情况,直接返回 `false`。 |
| 84 | + |
| 85 | +```javascript |
| 86 | +/** |
| 87 | + * Definition for a binary tree node. |
| 88 | + * function TreeNode(val) { |
| 89 | + * this.val = val; |
| 90 | + * this.left = this.right = null; |
| 91 | + * } |
| 92 | + */ |
| 93 | +/** |
| 94 | + * @param {TreeNode} root |
| 95 | + * @return {boolean} |
| 96 | + */ |
| 97 | +var isSymmetric = function (root) { |
| 98 | + if (!root) return true |
| 99 | + let queue = [root.left, root.right] |
| 100 | + while (queue.length) { |
| 101 | + let node1 = queue.shift() |
| 102 | + let node2 = queue.shift() |
| 103 | + if (!node1 && !node2) continue |
| 104 | + if (!node1 || !node2 || node1.val !== node2.val) return false |
| 105 | + queue.push(node1.left) |
| 106 | + queue.push(node2.right) |
| 107 | + queue.push(node1.right) |
| 108 | + queue.push(node2.left) |
| 109 | + } |
| 110 | + return true |
| 111 | +}; |
| 112 | +``` |
| 113 | + |
| 114 | +## 最后 |
| 115 | +文章产出不易,还望各位小伙伴们支持一波! |
| 116 | + |
| 117 | +往期精选: |
| 118 | + |
| 119 | +<a href="https://github.com/Chocolate1999/Front-end-learning-to-organize-notes">小狮子前端の笔记仓库</a> |
| 120 | + |
| 121 | +<a href="https://github.com/Chocolate1999/leetcode-javascript">leetcode-javascript:LeetCode 力扣的 JavaScript 解题仓库,前端刷题路线(思维导图)</a> |
| 122 | + |
| 123 | +小伙伴们可以在Issues中提交自己的解题代码,🤝 欢迎Contributing,可打卡刷题,Give a ⭐️ if this project helped you! |
| 124 | + |
| 125 | + |
| 126 | +<a href="https://yangchaoyi.vip/">访问超逸の博客</a>,方便小伙伴阅读玩耍~ |
| 127 | + |
| 128 | +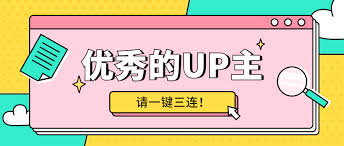 |
| 129 | + |
| 130 | +```javascript |
| 131 | +学如逆水行舟,不进则退 |
| 132 | +``` |
| 133 | + |
| 134 | + |
0 commit comments