|
| 1 | +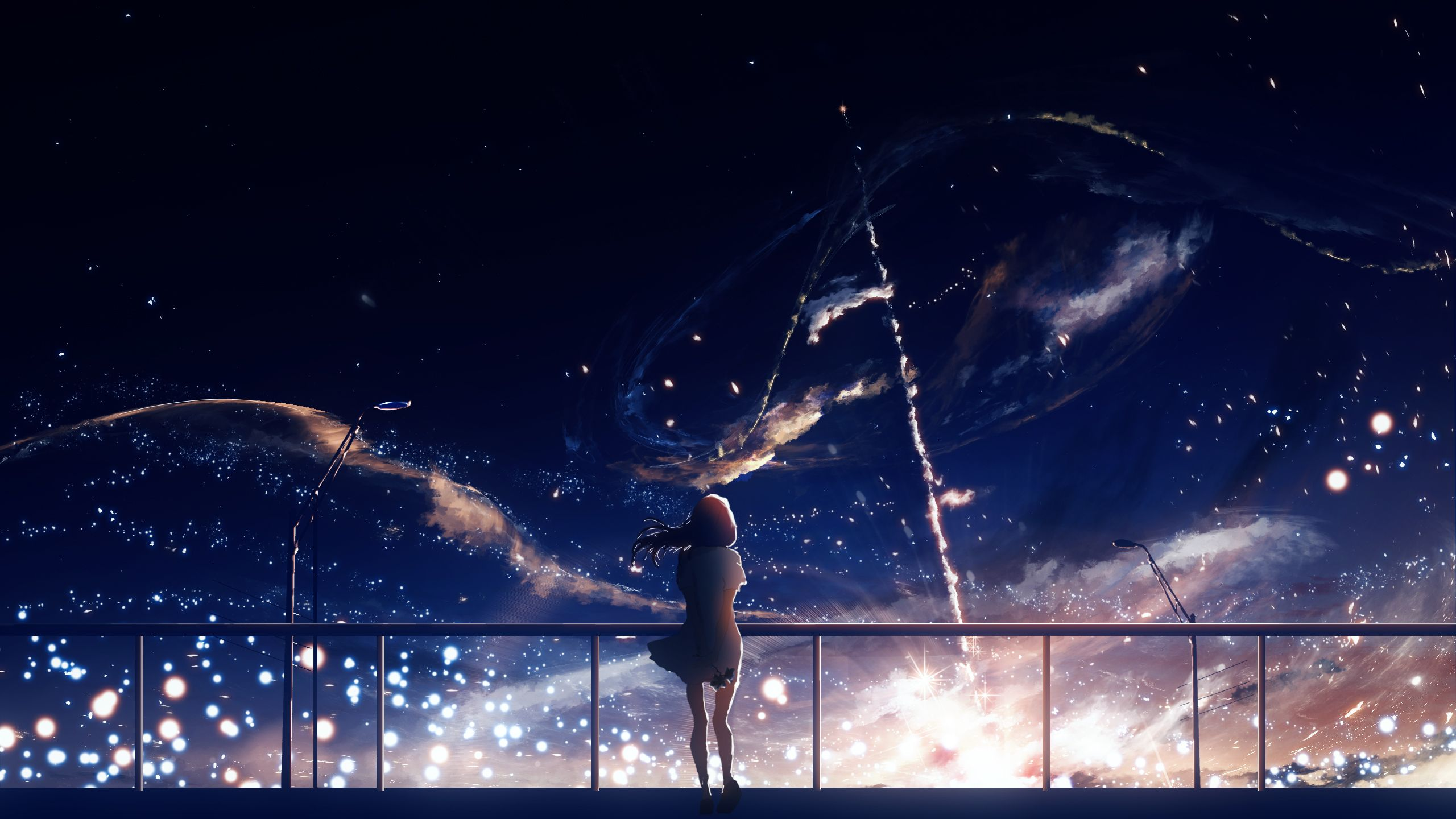 |
| 2 | +>仰望星空的人,不应该被嘲笑 |
| 3 | +
|
| 4 | +## 题目描述 |
| 5 | +给定一个非空二叉树,返回其最大路径和。 |
| 6 | + |
| 7 | +本题中,路径被定义为一条从树中任意节点出发,沿父节点-子节点连接,达到任意节点的序列。该路径至少包含一个节点,且不一定经过根节点。 |
| 8 | + |
| 9 | + |
| 10 | + |
| 11 | +示例 1: |
| 12 | + |
| 13 | +```javascript |
| 14 | +输入:[1,2,3] |
| 15 | + |
| 16 | + 1 |
| 17 | + / \ |
| 18 | + 2 3 |
| 19 | + |
| 20 | +输出:6 |
| 21 | +``` |
| 22 | + |
| 23 | +示例 2: |
| 24 | + |
| 25 | +```javascript |
| 26 | +输入:[-10,9,20,null,null,15,7] |
| 27 | + |
| 28 | + -10 |
| 29 | + / \ |
| 30 | + 9 20 |
| 31 | + / \ |
| 32 | + 15 7 |
| 33 | + |
| 34 | +输出:42 |
| 35 | +``` |
| 36 | + |
| 37 | +来源:力扣(LeetCode) |
| 38 | +链接:https://leetcode-cn.com/problems/binary-tree-maximum-path-sum |
| 39 | +著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。 |
| 40 | + |
| 41 | + |
| 42 | +## 解题思路 |
| 43 | +后序遍历,先遍历左孩子,对于孩子的累计和,我们判断一下,如果小于0(即为负数)就没必要加了,直接返回 0 即可,否则加上孩子累计和。然后我们对每一层求一下最大值即可。 |
| 44 | + |
| 45 | +```javascript |
| 46 | +/** |
| 47 | + * Definition for a binary tree node. |
| 48 | + * function TreeNode(val) { |
| 49 | + * this.val = val; |
| 50 | + * this.left = this.right = null; |
| 51 | + * } |
| 52 | + */ |
| 53 | +/** |
| 54 | + * @param {TreeNode} root |
| 55 | + * @return {number} |
| 56 | + */ |
| 57 | +var maxPathSum = function (root) { |
| 58 | + let res = Number.MIN_SAFE_INTEGER; |
| 59 | + let dfs = (root) => { |
| 60 | + if (!root) return 0; |
| 61 | + // 后序遍历,先遍历左孩子 |
| 62 | + let left = root.left && dfs(root.left); |
| 63 | + let right = root.right && dfs(root.right); |
| 64 | + // 每一层求一下最大值 |
| 65 | + res = Math.max(res, left + right + root.val); |
| 66 | + let sum = Math.max(left, right) + root.val; |
| 67 | + // 判断一下如果孩子的累计和小于0,就没必要加了 |
| 68 | + return sum < 0 ? 0 : sum; |
| 69 | + } |
| 70 | + dfs(root); |
| 71 | + return res; |
| 72 | +}; |
| 73 | +``` |
| 74 | + |
| 75 | +## 最后 |
| 76 | +文章产出不易,还望各位小伙伴们支持一波! |
| 77 | + |
| 78 | +往期精选: |
| 79 | + |
| 80 | +<a href="https://github.com/Chocolate1999/Front-end-learning-to-organize-notes">小狮子前端の笔记仓库</a> |
| 81 | + |
| 82 | +<a href="https://github.com/Chocolate1999/leetcode-javascript">leetcode-javascript:LeetCode 力扣的 JavaScript 解题仓库,前端刷题路线(思维导图)</a> |
| 83 | + |
| 84 | +小伙伴们可以在Issues中提交自己的解题代码,🤝 欢迎Contributing,可打卡刷题,Give a ⭐️ if this project helped you! |
| 85 | + |
| 86 | + |
| 87 | +<a href="https://yangchaoyi.vip/">访问超逸の博客</a>,方便小伙伴阅读玩耍~ |
| 88 | + |
| 89 | +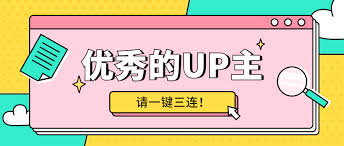 |
| 90 | + |
| 91 | +```javascript |
| 92 | +学如逆水行舟,不进则退 |
| 93 | +``` |
| 94 | + |
| 95 | + |
0 commit comments