Commit 81db134
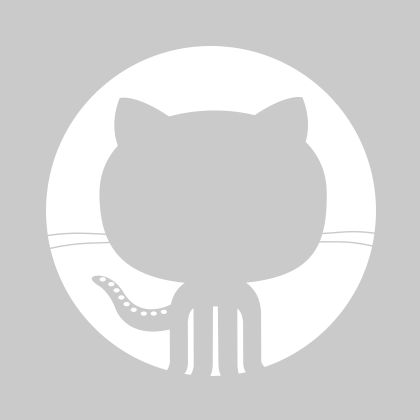
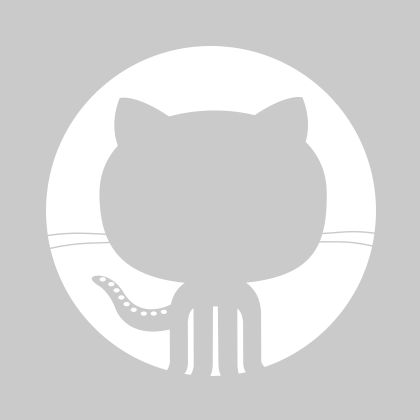
James Michael DuPont
James Michael DuPont
1 parent e3fb761 commit 81db134
File tree
6 files changed
+302
-180
lines changed6 files changed
+302
-180
lines changedLines changed: 177 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + | |
| 101 | + | |
| 102 | + | |
| 103 | + | |
| 104 | + | |
| 105 | + | |
| 106 | + | |
| 107 | + | |
| 108 | + | |
| 109 | + | |
| 110 | + | |
| 111 | + | |
| 112 | + | |
| 113 | + | |
| 114 | + | |
| 115 | + | |
| 116 | + | |
| 117 | + | |
| 118 | + | |
| 119 | + | |
| 120 | + | |
| 121 | + | |
| 122 | + | |
| 123 | + | |
| 124 | + | |
| 125 | + | |
| 126 | + | |
| 127 | + | |
| 128 | + | |
| 129 | + | |
| 130 | + | |
| 131 | + | |
| 132 | + | |
| 133 | + | |
| 134 | + | |
| 135 | + | |
| 136 | + | |
| 137 | + | |
| 138 | + | |
| 139 | + | |
| 140 | + | |
| 141 | + | |
| 142 | + | |
| 143 | + | |
| 144 | + | |
| 145 | + | |
| 146 | + | |
| 147 | + | |
| 148 | + | |
| 149 | + | |
| 150 | + | |
| 151 | + | |
| 152 | + | |
| 153 | + | |
| 154 | + | |
| 155 | + | |
| 156 | + | |
| 157 | + | |
| 158 | + | |
| 159 | + | |
| 160 | + | |
| 161 | + | |
| 162 | + | |
| 163 | + | |
| 164 | + | |
| 165 | + | |
| 166 | + | |
| 167 | + | |
| 168 | + | |
| 169 | + | |
| 170 | + | |
| 171 | + | |
| 172 | + | |
| 173 | + | |
| 174 | + | |
| 175 | + | |
| 176 | + | |
| 177 | + |
Lines changed: 14 additions & 131 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
13 | 13 |
| |
14 | 14 |
| |
15 | 15 |
| |
16 |
| - | |
17 | 16 |
| |
18 | 17 |
| |
19 | 18 |
| |
| |||
24 | 23 |
| |
25 | 24 |
| |
26 | 25 |
| |
27 |
| - | |
28 |
| - | |
29 |
| - | |
30 |
| - | |
31 |
| - | |
32 |
| - | |
33 |
| - | |
34 |
| - | |
35 |
| - | |
36 |
| - | |
37 |
| - | |
38 |
| - | |
39 |
| - | |
40 |
| - | |
41 |
| - | |
42 |
| - | |
43 |
| - | |
44 |
| - | |
45 |
| - | |
46 |
| - | |
47 |
| - | |
48 |
| - | |
49 |
| - | |
50 |
| - | |
51 |
| - | |
52 |
| - | |
53 |
| - | |
54 |
| - | |
55 |
| - | |
56 |
| - | |
57 |
| - | |
58 |
| - | |
59 |
| - | |
60 |
| - | |
61 |
| - | |
62 |
| - | |
63 |
| - | |
64 |
| - | |
65 |
| - | |
66 |
| - | |
67 |
| - | |
68 |
| - | |
69 |
| - | |
70 |
| - | |
71 |
| - | |
72 |
| - | |
73 |
| - | |
74 |
| - | |
75 |
| - | |
76 |
| - | |
77 |
| - | |
78 |
| - | |
79 |
| - | |
80 |
| - | |
81 | 26 |
| |
| 27 | + | |
82 | 28 |
| |
83 |
| - | |
84 |
| - | |
85 |
| - | |
86 |
| - | |
87 |
| - | |
88 |
| - | |
89 |
| - | |
90 |
| - | |
91 |
| - | |
92 |
| - | |
93 |
| - | |
94 |
| - | |
95 |
| - | |
96 |
| - | |
97 |
| - | |
98 |
| - | |
99 |
| - | |
100 |
| - | |
101 |
| - | |
102 |
| - | |
103 |
| - | |
104 |
| - | |
105 |
| - | |
106 |
| - | |
107 |
| - | |
108 |
| - | |
109 |
| - | |
110 |
| - | |
111 |
| - | |
112 |
| - | |
113 |
| - | |
114 |
| - | |
115 |
| - | |
116 |
| - | |
117 |
| - | |
118 |
| - | |
119 |
| - | |
120 |
| - | |
121 |
| - | |
122 |
| - | |
123 |
| - | |
124 |
| - | |
125 |
| - | |
126 |
| - | |
127 |
| - | |
128 |
| - | |
129 |
| - | |
130 |
| - | |
131 |
| - | |
132 |
| - | |
133 |
| - | |
134 |
| - | |
135 |
| - | |
136 |
| - | |
137 |
| - | |
138 |
| - | |
139 |
| - | |
140 |
| - | |
141 |
| - | |
142 |
| - | |
143 |
| - | |
144 |
| - | |
145 |
| - | |
146 |
| - | |
147 |
| - | |
148 |
| - | |
149 |
| - | |
150 |
| - | |
151 |
| - | |
152 |
| - | |
153 |
| - | |
154 |
| - | |
155 |
| - | |
156 |
| - | |
157 |
| - | |
158 |
| - | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
159 | 42 |
| |
160 | 43 |
| |
161 | 44 |
|
Lines changed: 15 additions & 5 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 |
| - | |
| 2 | + | |
3 | 3 |
| |
4 | 4 |
| |
5 | 5 |
| |
6 | 6 |
| |
7 | 7 |
| |
8 | 8 |
| |
9 | 9 |
| |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
10 | 14 |
| |
11 | 15 |
| |
12 | 16 |
| |
| |||
116 | 120 |
| |
117 | 121 |
| |
118 | 122 |
| |
119 |
| - | |
120 |
| - | |
| 123 | + | |
| 124 | + | |
| 125 | + | |
121 | 126 |
| |
122 | 127 |
| |
123 | 128 |
| |
124 | 129 |
| |
125 | 130 |
| |
126 | 131 |
| |
127 | 132 |
| |
128 |
| - | |
129 |
| - | |
| 133 | + | |
| 134 | + | |
| 135 | + | |
130 | 136 |
| |
| 137 | + | |
| 138 | + | |
131 | 139 |
| |
| 140 | + | |
| 141 | + |
0 commit comments