|
| 1 | +# vue-double-list |
| 2 | + |
| 3 | +[ ](https://www.npmjs.com/package/vue-double-list) |
| 4 | +[](https://vuejs.org/) |
| 5 | + |
| 6 | +Double List Selection Component for Vue.js |
| 7 | + |
| 8 | +[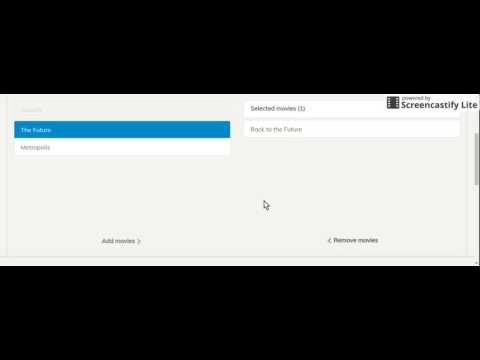](https://www.youtube.com/watch?v=qkYWaaMLCBM) |
| 9 | + |
| 10 | +## Table of contents |
| 11 | + |
| 12 | +- [Installation](#installation) |
| 13 | +- [Usage](#usage) |
| 14 | +- [Example](#example) |
| 15 | + |
| 16 | +# Installation |
| 17 | + |
| 18 | +``` |
| 19 | +npm install --save vue-double-list |
| 20 | +``` |
| 21 | + |
| 22 | +## Default import |
| 23 | + |
| 24 | +```javascript |
| 25 | +import Vue from 'vue' |
| 26 | +import DoubleList from 'vue-double-list' |
| 27 | + |
| 28 | +Vue.use(DoubleList) |
| 29 | +``` |
| 30 | + |
| 31 | +**⚠️ A css file is included when importing the package. You may have to setup your bundler to embed the css in your page.** |
| 32 | + |
| 33 | +## Browser |
| 34 | + |
| 35 | +```html |
| 36 | +<link rel="stylesheet" href="vue-double-list/dist/vue-double-list.css"/> |
| 37 | + |
| 38 | +<script src="vue.js"></script> |
| 39 | +<script src="vue-double-list/dist/vue-double-list.js"></script> |
| 40 | +``` |
| 41 | + |
| 42 | +The plugin should be auto-installed. If not, you can install it manually with the instructions below: |
| 43 | + |
| 44 | +```javascript |
| 45 | +Vue.use(DoubleList) |
| 46 | +``` |
| 47 | + |
| 48 | +# Usage |
| 49 | + |
| 50 | +```html |
| 51 | +<double-list label="movies" :items="listItems" v-model="selectedItems"></double-list> |
| 52 | +``` |
| 53 | + |
| 54 | +# Props |
| 55 | +| Name | Type | Default | Description | |
| 56 | +| ---:| --- | ---| --- | |
| 57 | +| items | Array | [] | List items for selection | |
| 58 | +| label | String | "" | Name describing items, used in UI for buttons, labels etc. | |
| 59 | +| itemFilterKey | String | undefined | If items are objects, filter list on this item property | |
| 60 | +| itemIdKey | String | undefined | If items are objects, use this item property for object identity | |
| 61 | +| value | Array | [] | List of selected items, updated on @input | |
| 62 | + |
| 63 | +# Example |
| 64 | + |
| 65 | +With plain list items: |
| 66 | + |
| 67 | +```javascript |
| 68 | +new Vue({ |
| 69 | + el: '#vue', |
| 70 | + methods: { |
| 71 | + onInput: function() { |
| 72 | + console.log('Selection changed', this.selectedItems); |
| 73 | + } |
| 74 | + }, |
| 75 | + data: { |
| 76 | + listItems: ['Back to the Future', 'The Future', 'Metropolis'], |
| 77 | + selectedItems: [] |
| 78 | + } |
| 79 | +}) |
| 80 | +``` |
| 81 | + |
| 82 | +With object list items, will keep selection by item's id when listItems is updated: |
| 83 | + |
| 84 | +```javascript |
| 85 | +new Vue({ |
| 86 | + el: '#vue', |
| 87 | + data: { |
| 88 | + listItems: [ |
| 89 | + {id: 1, title: 'Back to the Future'}, |
| 90 | + {id: 2, title: 'The Future'}, |
| 91 | + {id: 3, title: 'Metropolis'} |
| 92 | + ], |
| 93 | + selectedItems: [] |
| 94 | + } |
| 95 | +}) |
| 96 | +``` |
| 97 | + |
| 98 | +To customize list item rendering, use named slot: |
| 99 | + |
| 100 | +```html |
| 101 | +<double-list label="movies" :items="listItems" v-model="selectedItems"> |
| 102 | +<template slot="item" scope="props"> |
| 103 | + {{ props.item.title }} |
| 104 | +</template> |
| 105 | +</double-list> |
| 106 | +``` |
| 107 | + |
| 108 | +--- |
| 109 | + |
| 110 | +# Plugin Development |
| 111 | + |
| 112 | +## Installation |
| 113 | + |
| 114 | +The first time you create or clone your plugin, you need to install the default dependencies: |
| 115 | + |
| 116 | +``` |
| 117 | +npm install |
| 118 | +``` |
| 119 | + |
| 120 | +## Watch and compile |
| 121 | + |
| 122 | +This will run webpack in watching mode and output the compiled files in the `dist` folder. |
| 123 | + |
| 124 | +``` |
| 125 | +npm run dev |
| 126 | +``` |
| 127 | + |
| 128 | +## Use it in another project |
| 129 | + |
| 130 | +While developping, you can follow the install instructions of your plugin and link it into the project that uses it. |
| 131 | + |
| 132 | +In the plugin folder: |
| 133 | + |
| 134 | +``` |
| 135 | +npm link |
| 136 | +``` |
| 137 | + |
| 138 | +In the other project folder: |
| 139 | + |
| 140 | +``` |
| 141 | +npm link vue-double-list |
| 142 | +``` |
| 143 | + |
| 144 | +This will install it in the dependencies as a symlink, so that it gets any modifications made to the plugin. |
| 145 | + |
| 146 | +## Publish to npm |
| 147 | + |
| 148 | +You may have to login to npm before, with `npm adduser`. The plugin will be built in production mode before getting published on npm. |
| 149 | + |
| 150 | +``` |
| 151 | +npm publish |
| 152 | +``` |
| 153 | + |
| 154 | +## Manual build |
| 155 | + |
| 156 | +This will build the plugin into the `dist` folder in production mode. |
| 157 | + |
| 158 | +``` |
| 159 | +npm run build |
| 160 | +``` |
| 161 | + |
| 162 | +--- |
| 163 | + |
| 164 | +## License |
| 165 | + |
| 166 | +[MIT](http://opensource.org/licenses/MIT) |
0 commit comments