-
-
Notifications
You must be signed in to change notification settings - Fork 34
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
5 changed files
with
220 additions
and
1 deletion.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,136 @@ | ||
// LiteLoaderScript Dev Helper | ||
/// <reference path="d:\Coding\LLSEAids/dts/llaids/src/index.d.ts"/> | ||
/* global logger ll mc logger */ | ||
|
||
const { existsSync, mkdirSync, writeFileSync, readFileSync } = require('fs'); | ||
const { resolve, join } = require('path'); | ||
const checkDiskSpace = require('check-disk-space').default; | ||
|
||
const config = { | ||
checkInterval: 60, | ||
percent: 0.95, | ||
stopInterval: 20, | ||
showDiskLog: true, | ||
}; | ||
|
||
const pluginName = 'DiskUsageStop'; | ||
const pluginVer = [0, 1, 0]; | ||
|
||
const pluginDataPath = `plugins/${pluginName}`; | ||
const pluginConfigPath = join(pluginDataPath, 'config.json'); | ||
if (!existsSync(pluginDataPath)) mkdirSync(pluginDataPath); | ||
|
||
logger.setTitle(pluginName); | ||
|
||
function formatError(e) { | ||
let msg = e; | ||
if (e instanceof Error) msg = e.stack || e.message; | ||
return String(msg); | ||
} | ||
|
||
function logError(e) { | ||
logger.error(`插件出错!\n${formatError(e)}`); | ||
} | ||
|
||
function reloadConfigSync() { | ||
if (!existsSync(pluginConfigPath)) { | ||
logger.warn(`插件配置文件不存在,已写入默认配置到 ${pluginConfigPath}`); | ||
writeFileSync(pluginConfigPath, JSON.stringify(config, undefined, 2), { | ||
encoding: 'utf-8', | ||
}); | ||
} else { | ||
let loadedConf; | ||
try { | ||
loadedConf = JSON.parse( | ||
readFileSync(pluginConfigPath, { encoding: 'utf-8' }) | ||
); | ||
} catch (e) { | ||
logger.error(`读取插件配置失败,将使用默认配置\n${formatError(e)}`); | ||
return; | ||
} | ||
|
||
Object.entries(loadedConf).forEach((e) => { | ||
const [k, v] = e; | ||
config[k] = v; | ||
}); | ||
} | ||
} | ||
|
||
/** | ||
* @param {(...args:any[])=>Promise<Any>} func | ||
*/ | ||
function warpAsyncFunction(func) { | ||
return (...args) => { | ||
func(...args).catch(logError); | ||
}; | ||
} | ||
|
||
/** | ||
* @param {number} space | ||
* @param {number} pointLength | ||
* @returns | ||
*/ | ||
function formatSpace(space, pointLength = 2) { | ||
const units = ['B', 'K', 'M', 'G']; | ||
let currentUnit = units.shift(); | ||
while (units.length > 0 && space >= 1024) { | ||
currentUnit = units.shift(); | ||
space /= 1024; | ||
} | ||
return `${space.toFixed(pointLength)}${currentUnit}`; | ||
} | ||
|
||
mc.listen('onServerStarted', () => { | ||
const checkTask = async () => { | ||
const { stopInterval, percent, showDiskLog } = config; | ||
const { free, size } = await checkDiskSpace(resolve(__dirname)); | ||
|
||
const usedSpace = size - free; | ||
const usedPercent = usedSpace / size; | ||
|
||
const formattedUsed = formatSpace(usedSpace); | ||
const formattedTotal = formatSpace(size); | ||
const formattedPercent = (usedPercent * 100).toFixed(2); | ||
|
||
if (showDiskLog) | ||
logger.info( | ||
`[定时监测] 磁盘已用 ${formattedUsed} / ${formattedTotal} (${formattedPercent}%)` | ||
); | ||
|
||
if (usedPercent >= percent) { | ||
logger.warn('磁盘使用达到限制,即将关服'); | ||
|
||
const toastTitle = | ||
`§g服务器存储占用已到达 §c§l${formattedPercent}%%§r ` + | ||
`§7(§e${formattedUsed} §7/ §6${formattedTotal}§7)§g!`; | ||
const toastMsg = `§g将在 §d§l${stopInterval} §r§g秒后关闭服务器!`; | ||
mc.getOnlinePlayers().forEach((p) => p.sendToast(toastTitle, toastMsg)); | ||
mc.broadcast(`${toastTitle}\n${toastMsg}`); | ||
|
||
let countdown = stopInterval; | ||
const stopTask = setInterval(() => { | ||
countdown -= 1; | ||
if (countdown <= 0) { | ||
mc.runcmd('stop'); | ||
clearInterval(stopTask); | ||
} else { | ||
mc.broadcast(`§g还有 §d§l${countdown} §r§g秒关闭服务器!`); | ||
} | ||
}, 1000); | ||
} | ||
}; | ||
|
||
setInterval(warpAsyncFunction(checkTask), config.checkInterval * 1000); | ||
checkTask(); | ||
}); | ||
|
||
reloadConfigSync(); | ||
ll.registerPlugin( | ||
pluginName, | ||
'当你BDS所在硬盘分区使用量超过指定比例时停服', | ||
pluginVer, | ||
{ | ||
Author: 'student_2333', | ||
License: 'Apache-2.0', | ||
} | ||
); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,22 @@ | ||
{ | ||
"name": "disk-usage-stop", | ||
"version": "0.1.0", | ||
"description": "A LLSE plugin stops your server when the current disk part usage attaches the limit", | ||
"main": "index.cjs", | ||
"scripts": { | ||
"test": "echo \"Error: no test specified\" && exit 1" | ||
}, | ||
"repository": { | ||
"type": "git", | ||
"url": "git+https://github.com/lgc2333/LLSEPlugins.git" | ||
}, | ||
"author": "student_2333 <[email protected]>", | ||
"license": "Apache-2.0", | ||
"bugs": { | ||
"url": "https://github.com/lgc2333/LLSEPlugins/issues" | ||
}, | ||
"homepage": "https://github.com/lgc2333/LLSEPlugins#readme", | ||
"dependencies": { | ||
"check-disk-space": "^3.3.1" | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,59 @@ | ||
<!-- markdownlint-disable MD036 MD033 --> | ||
|
||
# DiskUsageStop | ||
|
||
当你 BDS 所在硬盘分区使用量超过指定比例时停服 | ||
|
||
下载插件请去 [Releases](https://github.com/lgc2333/LLSEPlugins/releases) | ||
|
||
_妈的,插件撞车了,但我还是给它写出来了,喜欢用哪个就用哪个吧_ | ||
|
||
# 介绍 | ||
|
||
功能不多,看截图 | ||
|
||
 | ||
|
||
# 配置文件 | ||
|
||
配置文件路径 `plugins/DiskUsageStop/config.json`,初次加载插件会自动生成 | ||
请注意实际的配置文件不能有注释 | ||
|
||
```jsonc | ||
{ | ||
// 检查硬盘容量的时间间隔(秒) | ||
"checkInterval": 60, | ||
|
||
// 当硬盘已用空间比例达到此值时关闭服务器 | ||
// 0.95 为 95% | ||
"percent": 0.95, | ||
|
||
// 检测到硬盘已用空间已到指定比例时倒数关服的时长(秒) | ||
"stopInterval": 20, | ||
|
||
// 检测硬盘容量时是否在控制台输出信息 | ||
"showDiskLog": true | ||
} | ||
``` | ||
|
||
## 联系我 | ||
|
||
QQ:3076823485 | ||
吹水群:[1105946125](https://jq.qq.com/?_wv=1027&k=Z3n1MpEp) | ||
邮箱:<[email protected]> | ||
|
||
## 赞助 | ||
|
||
感谢大家的赞助!你们的赞助将是我继续创作的动力! | ||
|
||
- [爱发电](https://afdian.net/@lgc2333) | ||
- <details> | ||
<summary>赞助二维码(点击展开)</summary> | ||
|
||
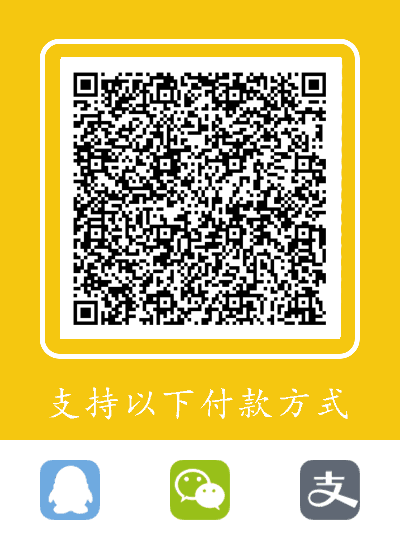 | ||
|
||
</details> | ||
|
||
## 更新日志 | ||
|
||
暂无 |
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.