Commit 53daec5
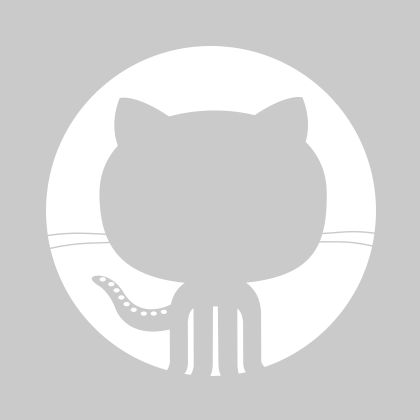
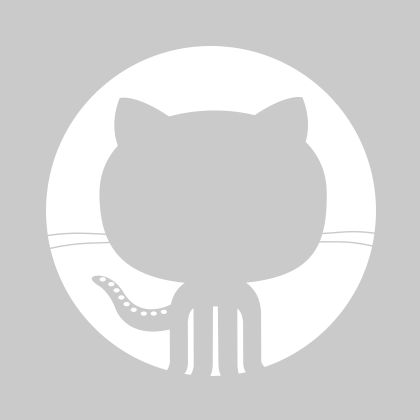
Stefan Rogin
Stefan Rogin
1 parent 9d040c6 commit 53daec5
File tree
6 files changed
+239
-36
lines changed- examples
- messagebird
- tests
6 files changed
+239
-36
lines changedLines changed: 26 additions & 30 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 |
| - | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
4 | 6 |
| |
5 | 7 |
| |
6 | 8 |
| |
7 | 9 |
| |
8 |
| - | |
9 |
| - | |
| 10 | + | |
| 11 | + | |
10 | 12 |
| |
11 | 13 |
| |
12 |
| - | |
| 14 | + | |
13 | 15 |
| |
14 |
| - | |
15 |
| - | |
| 16 | + | |
| 17 | + | |
16 | 18 |
| |
17 | 19 |
| |
18 |
| - | |
| 20 | + | |
19 | 21 |
| |
20 |
| - | |
21 |
| - | |
| 22 | + | |
| 23 | + | |
22 | 24 |
| |
23 | 25 |
| |
24 |
| - | |
25 |
| - | |
26 |
| - | |
27 |
| - | |
28 |
| - | |
29 |
| - | |
30 |
| - | |
31 |
| - | |
32 |
| - | |
33 |
| - | |
34 |
| - | |
35 |
| - | |
36 |
| - | |
37 |
| - | |
38 |
| - | |
39 |
| - | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
40 | 36 |
| |
41 |
| - | |
| 37 | + | |
42 | 38 |
| |
43 |
| - | |
44 |
| - | |
45 |
| - | |
46 |
| - | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + |
Lines changed: 70 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + |
Lines changed: 6 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
19 | 19 |
| |
20 | 20 |
| |
21 | 21 |
| |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + |
Lines changed: 9 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
45 | 45 |
| |
46 | 46 |
| |
47 | 47 |
| |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + |
Lines changed: 23 additions & 4 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
124 | 124 |
| |
125 | 125 |
| |
126 | 126 |
| |
| 127 | + | |
| 128 | + | |
| 129 | + | |
| 130 | + | |
| 131 | + | |
| 132 | + | |
| 133 | + | |
| 134 | + | |
| 135 | + | |
| 136 | + | |
| 137 | + | |
| 138 | + | |
| 139 | + | |
| 140 | + | |
| 141 | + | |
| 142 | + | |
| 143 | + | |
| 144 | + | |
| 145 | + | |
127 | 146 |
| |
128 | 147 |
| |
129 | 148 |
| |
| |||
155 | 174 |
| |
156 | 175 |
| |
157 | 176 |
| |
158 |
| - | |
| 177 | + | |
159 | 178 |
| |
160 | 179 |
| |
161 | 180 |
| |
162 |
| - | |
| 181 | + | |
163 | 182 |
| |
164 | 183 |
| |
165 | 184 |
| |
| |||
169 | 188 |
| |
170 | 189 |
| |
171 | 190 |
| |
172 |
| - | |
173 |
| - | |
| 191 | + | |
| 192 | + | |
174 | 193 |
| |
175 | 194 |
| |
176 | 195 |
| |
|
Lines changed: 105 additions & 2 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
1 | 2 |
| |
2 | 3 |
| |
| 4 | + | |
3 | 5 |
| |
4 | 6 |
| |
5 | 7 |
| |
| |||
13 | 15 |
| |
14 | 16 |
| |
15 | 17 |
| |
16 |
| - | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
17 | 43 |
| |
18 | 44 |
| |
19 | 45 |
| |
20 | 46 |
| |
21 | 47 |
| |
22 |
| - | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
| 88 | + | |
| 89 | + | |
| 90 | + | |
| 91 | + | |
| 92 | + | |
| 93 | + | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + | |
| 101 | + | |
| 102 | + | |
| 103 | + | |
| 104 | + | |
| 105 | + | |
| 106 | + | |
| 107 | + | |
| 108 | + | |
| 109 | + | |
| 110 | + | |
| 111 | + | |
| 112 | + | |
| 113 | + | |
| 114 | + | |
| 115 | + | |
| 116 | + | |
| 117 | + | |
| 118 | + | |
| 119 | + | |
| 120 | + | |
| 121 | + | |
| 122 | + | |
| 123 | + | |
| 124 | + | |
| 125 | + |
0 commit comments