This library provides the implementation for the RevocationList2020
proposal described here.
The following demo uses the wasm library on a web page.
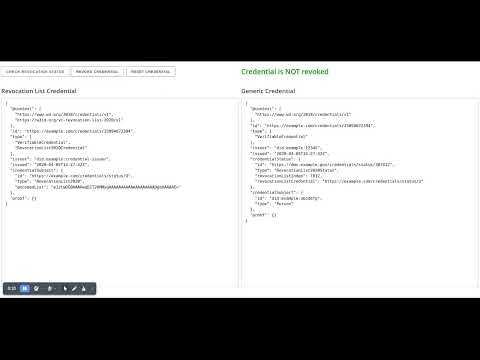
To run the demo locally, build the javascript library using:
wasm-pack build --target web --debug
and then launch a local web server, for example using python:
python3 -m http.server 7654
The following example shows how to use the library with Rust.
|
println!("Hello, RevocationList2020!"); |
|
|
|
// create a new revocation list |
|
let mut rl = RevocationList2020::new("https://example.com/credentials/status/3", 16).unwrap(); |
|
println!("{}", rl); |
|
|
|
// create a credential that uses the revocation list |
|
let c = create_credential(); |
|
let c_idx = c.credential_status.revocation_list_index; |
|
|
|
// check if the credential is revoked |
|
let revoked = rl.is_revoked(&c).unwrap(); |
|
println!("credential at index {} is revoked? {}", c_idx, revoked); |
|
// revoke the credential |
|
println!("revoking credential at index {}", c_idx); |
|
rl.revoke(&c).unwrap(); |
|
// print the updated revocation list |
|
println!("{}", rl); |
|
//check if the credential is revoked |
|
let revoked = rl.is_revoked(&c).unwrap(); |
|
println!("credential at index {} is revoked? {}", c_idx, revoked); |
|
// reset the credential revocation |
|
println!("resetting status for credential at index {}", c_idx); |
|
rl.reset(&c).unwrap(); |
|
// print the updated revocation list |
|
println!("{}", rl); |
|
//check if the credential is revoked |
|
let revoked = rl.is_revoked(&c).unwrap(); |
|
println!("credential at index {} is revoked? {}", c_idx, revoked); |
To run the example locally use the command:
To run tests, run the following command
The rust library is published on crates.io
The wasm library is published on npmjs