@@ -204,6 +204,78 @@ keymaps = {
204
204
}
205
205
` ` `
206
206
207
+ # ## Use `vim.ui.input()`
208
+
209
+ This example shows how to use the Neovim builtin ` vim.ui.input()` to create a
210
+ simple input prompt, and create a new commit with the user' s given message.
211
+
212
+ 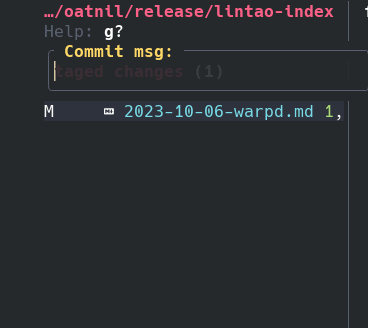
213
+
214
+ For Neovim ≥ v0.10:
215
+
216
+ ```lua
217
+ keymaps = {
218
+ file_panel = {
219
+ {
220
+ "n", "cc",
221
+ function()
222
+ vim.ui.input({ prompt = "Commit message: " }, function(msg)
223
+ if not msg then return end
224
+ local results = vim.system({ "git", "commit", "-m", msg }, { text = true }):wait()
225
+
226
+ if results.code ~= 0 then
227
+ vim.notify(
228
+ "Commit failed with the message: \n"
229
+ .. vim.trim(results.stdout .. "\n" .. results.stderr),
230
+ vim.log.levels.ERROR,
231
+ { title = "Commit" }
232
+ )
233
+ else
234
+ vim.notify(results.stdout, vim.log.levels.INFO, { title = "Commit" })
235
+ end
236
+ end)
237
+ end,
238
+ },
239
+ },
240
+ }
241
+ ```
242
+
243
+ <details>
244
+ <summary><b>For Neovim ≤ v0.10, use this function in place of <code>vim.system()</code></b></summary>
245
+
246
+ ```lua
247
+ --- @class system.Results
248
+ --- @field code integer
249
+ --- @field stdout string
250
+ --- @field stderr string
251
+
252
+ --- @param cmd string|string[]
253
+ --- @return system.Results
254
+ local function system(cmd)
255
+ local results = {}
256
+
257
+ local function callback(_, data, event)
258
+ if event == "exit" then results.code = data
259
+ elseif event == "stdout" or event == "stderr" then
260
+ results[event] = table.concat(data, "\n")
261
+ end
262
+ end
263
+
264
+ vim.fn.jobwait({
265
+ vim.fn.jobstart(cmd, {
266
+ on_exit = callback,
267
+ on_stdout = callback,
268
+ on_stderr = callback,
269
+ stdout_buffered = true,
270
+ stderr_buffered = true,
271
+ })
272
+ })
273
+
274
+ return results
275
+ end
276
+ ```
277
+ </details>
278
+
207
279
### Use `:!cmd`
208
280
209
281
If you only ever write simple commit messages you could make use of `:h !cmd`:
0 commit comments