-
Notifications
You must be signed in to change notification settings - Fork 76
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Browse files
Browse the repository at this point in the history
<!-- Please precisely, concisely, and concretely describe what this PR changes, the rationale behind codes, and how it affects the users and other developers. --> ### This PR resolves [#3013](#3013) issue **feature:** - Add container commit functionality to the session detail panel. - The image name is a combination of alphabets, numbers, -, and _ from 4 to 32 characters. **How to test:** - Connect to an environment that supports image commit - Enable image commit in config.toml and create a session with a non-system role. - Access the session detail panel and click the commit feature in the top right corner. You can access via query string (?sessionDetail=<sessionID>, or endpoint detail page). - Set a session name and click the OK button. - Verify that the notification tracks the background task properly - Verify that the commit image is registered properly. 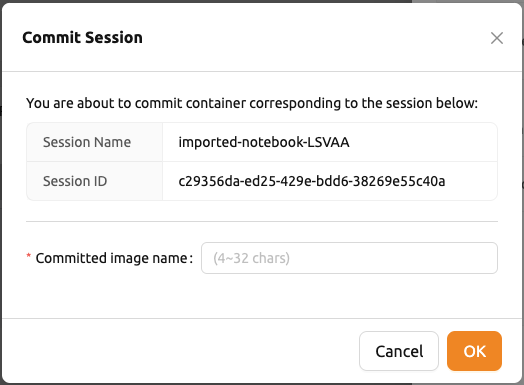 **Checklist:** (if applicable) - [ ] Mention to the original issue - [ ] Documentation - [ ] Minium required manager version - [ ] Specific setting for review (eg., KB link, endpoint or how to setup) - [ ] Minimum requirements to check during review - [ ] Test case(s) to demonstrate the difference of before/after
- Loading branch information
1 parent
38b29bd
commit 1efdc0c
Showing
25 changed files
with
231 additions
and
36 deletions.
There are no files selected for viewing
141 changes: 141 additions & 0 deletions
141
react/src/components/ComputeSessionNodeItems/ContainerCommitModal.tsx
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,141 @@ | ||
import { useSuspendedBackendaiClient } from '../../hooks'; | ||
import { useSetBAINotification } from '../../hooks/useBAINotification'; | ||
import BAIModal, { BAIModalProps } from '../BAIModal'; | ||
import Flex from '../Flex'; | ||
import { ContainerCommitModalFragment$key } from './__generated__/ContainerCommitModalFragment.graphql'; | ||
import { | ||
Descriptions, | ||
Divider, | ||
Form, | ||
FormInstance, | ||
Input, | ||
Typography, | ||
} from 'antd'; | ||
import graphql from 'babel-plugin-relay/macro'; | ||
import { useRef, useState } from 'react'; | ||
import { useTranslation } from 'react-i18next'; | ||
import { useFragment } from 'react-relay'; | ||
|
||
interface ContainerCommitModalProps extends BAIModalProps { | ||
sessionFrgmt: ContainerCommitModalFragment$key | null; | ||
onRequestClose: () => void; | ||
} | ||
|
||
const ContainerCommitModal: React.FC<ContainerCommitModalProps> = ({ | ||
sessionFrgmt, | ||
onRequestClose, | ||
...modalProps | ||
}) => { | ||
const { t } = useTranslation(); | ||
const formRef = useRef<FormInstance>(null); | ||
const baiClient = useSuspendedBackendaiClient(); | ||
|
||
const { upsertNotification } = useSetBAINotification(); | ||
const [isConfirmLoading, setIsConfirmLoading] = useState<boolean>(false); | ||
|
||
const session = useFragment( | ||
graphql` | ||
fragment ContainerCommitModalFragment on ComputeSessionNode { | ||
id | ||
name | ||
row_id @required(action: NONE) | ||
} | ||
`, | ||
sessionFrgmt, | ||
); | ||
|
||
const convertSessionToImage = () => { | ||
setIsConfirmLoading(true); | ||
formRef?.current | ||
?.validateFields() | ||
.then((values: { imageName: string }) => { | ||
baiClient.computeSession | ||
.convertSessionToImage(session?.name ?? '', values.imageName) | ||
.then((res: { task_id: string }) => { | ||
onRequestClose(); | ||
upsertNotification({ | ||
key: 'commitSession:' + session?.name, | ||
backgroundTask: { | ||
taskId: res.task_id, | ||
status: 'pending', | ||
statusDescriptions: { | ||
pending: t('session.CommitOnGoing'), | ||
resolved: t('session.CommitFinished'), | ||
rejected: t('session.CommitFailed'), | ||
}, | ||
}, | ||
duration: 0, | ||
message: 'commitSession: ' + session?.name, | ||
open: true, | ||
}); | ||
}) | ||
.catch((err: any) => { | ||
if (err?.message) { | ||
throw new Error(err.message); | ||
} else { | ||
throw err; | ||
} | ||
}) | ||
.finally(() => { | ||
setIsConfirmLoading(false); | ||
}); | ||
}) | ||
.catch((err) => { | ||
console.log(err); | ||
}); | ||
}; | ||
|
||
return ( | ||
<BAIModal | ||
title={t('session.CommitSession')} | ||
onOk={() => convertSessionToImage()} | ||
okButtonProps={{ loading: isConfirmLoading }} | ||
onCancel={onRequestClose} | ||
{...modalProps} | ||
destroyOnClose | ||
> | ||
<Flex | ||
direction="column" | ||
gap={'xs'} | ||
align="stretch" | ||
style={{ overflow: 'hidden' }} | ||
> | ||
<Typography.Text>{t('session.DescCommitSession')}</Typography.Text> | ||
<Descriptions bordered size="small" column={1}> | ||
<Descriptions.Item label={t('session.SessionName')}> | ||
{session?.name} | ||
</Descriptions.Item> | ||
<Descriptions.Item label={t('session.SessionId')}> | ||
{session?.row_id} | ||
</Descriptions.Item> | ||
{/* FIXME: need to use legacy_session */} | ||
{/* <Descriptions.Item label={t('session.launcher.Environments')}> | ||
</Descriptions.Item> */} | ||
</Descriptions> | ||
<Divider style={{ marginTop: 12, marginBottom: 12 }} /> | ||
<Form ref={formRef}> | ||
<Form.Item | ||
label={t('session.CommitImageName')} | ||
name="imageName" | ||
required | ||
rules={[ | ||
{ required: true }, | ||
{ | ||
min: 4, | ||
max: 32, | ||
}, | ||
{ | ||
pattern: /^[a-zA-Z0-9-_.]+$/, | ||
message: t('session.Validation.EnterValidSessionName'), | ||
}, | ||
]} | ||
> | ||
<Input placeholder={t('inputLimit.4to32chars')} /> | ||
</Form.Item> | ||
</Form> | ||
</Flex> | ||
</BAIModal> | ||
); | ||
}; | ||
|
||
export default ContainerCommitModal; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.