-
Notifications
You must be signed in to change notification settings - Fork 76
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Browse files
Browse the repository at this point in the history
resolves #2861 ([FR-255](https://lablup.atlassian.net/browse/FR-255?atlOrigin=eyJpIjoiODA5YzI1OGQ2OWJhNGM5NThmMWFmNWU3NWVhY2QwZjYiLCJwIjoiaiJ9)) Adds file attachment synchronization support to the LLM chat interface, enabling consistent file handling across multiple chat instances. When files are attached in one chat window, they are now properly synchronized across all active chat sessions. **Changes:** - Introduces `synchronizedAttachmentState` atom for managing shared file attachments - Adds file attachment handling in `EndpointLLMChatCard` - Implements `createDataTransferFiles` utility function for consistent file processing - Updates attachment state management in `LLMChatCard` **Checklist:** - [ ] Documentation - [ ] Minium required manager version - [x] Specific setting for review: - Test file attachments in synchronized chat mode - Verify attachments persist across chat instances - [x] Minimum requirements to check during review: - File attachments sync correctly between chat windows - Attachments clear properly after submission - Supported file types (image/*, text/*) work as expected - [x] Test cases: 1. Attach files in one chat window, verify sync in others 2. Submit message with attachments, confirm proper clearing 3. Test with various file types to ensure compatibility **Screenshots:** 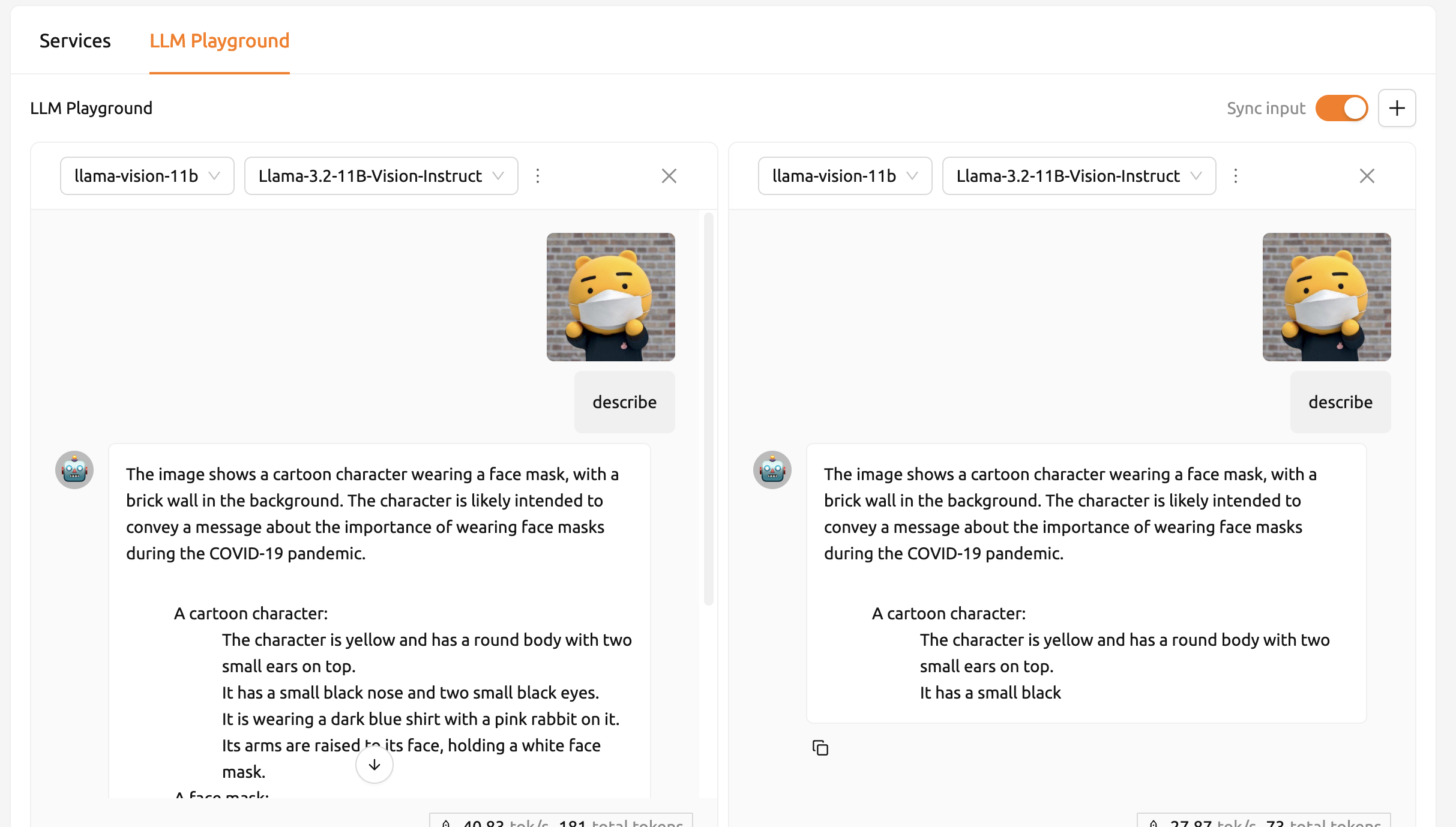] [FR-255]: https://lablup.atlassian.net/browse/FR-255?atlOrigin=eyJpIjoiNWRkNTljNzYxNjVmNDY3MDlhMDU5Y2ZhYzA5YTRkZjUiLCJwIjoiZ2l0aHViLWNvbS1KU1cifQ
- Loading branch information
Showing
3 changed files
with
70 additions
and
23 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters