-
Notifications
You must be signed in to change notification settings - Fork 710
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Add remaining schema renderers (#5534)
### Description of the change In #5436 we mentioned that several fields were still pending, namely - 1st level: object (at least, as array of tuples <string, any> for defining each property. - Array level: enum, arrays, objects WRT the top-level objects, it is not an actual problem: if they have `properties`, they will get rendered as any other nested param more. It is only the case when it lacks the `properties` fields that we need to handle. - Solution: allow any serialized object like `{"foo": 1234}` and add more validation messages. WRT the array-level properties: - Solution for enum: use the same approach as in the top-level enum. - Solution for arrays and objects: use the same approach as in the top-level objects: render a text field but enforce validation. Additionally, the array now enforces `maxItems` and `minItems` ### Benefits Every json schema datatype is now supported. ### Possible drawbacks N/A ### Applicable issues - fixes #5436 ### Additional information > **Note** > This PR is part of a series of PRs aimed at closing [this milestone](https://github.com/vmware-tanzu/kubeapps/milestone/27). I have split the changes to ease the review process, but as there are many interrelated changes, the tests will be performed in a separate PR (on top of the branch containing all the changes). > PR 5 out of 6 #### `Top-level objects`: 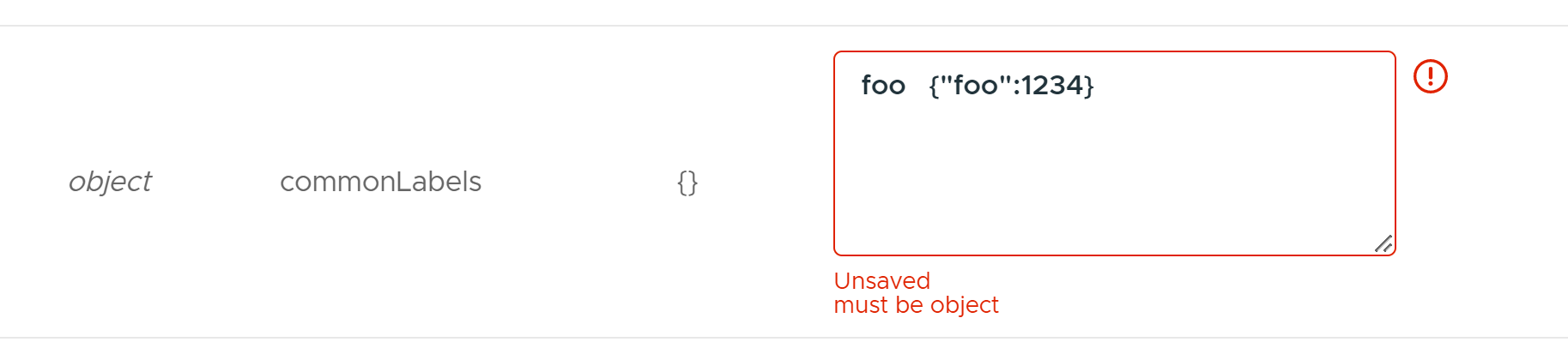 #### `Array<enum>`: 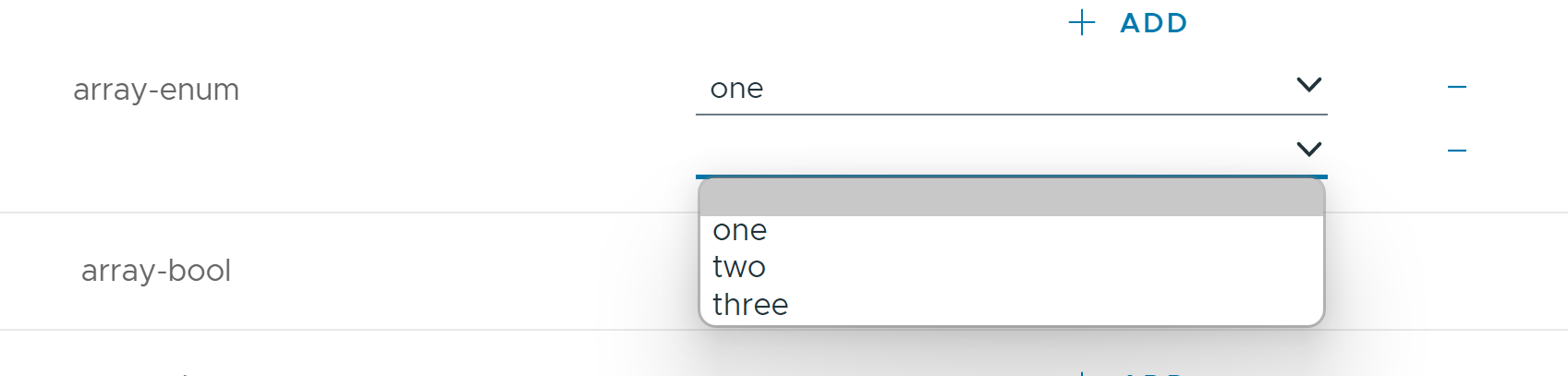 #### `Array<array>`: 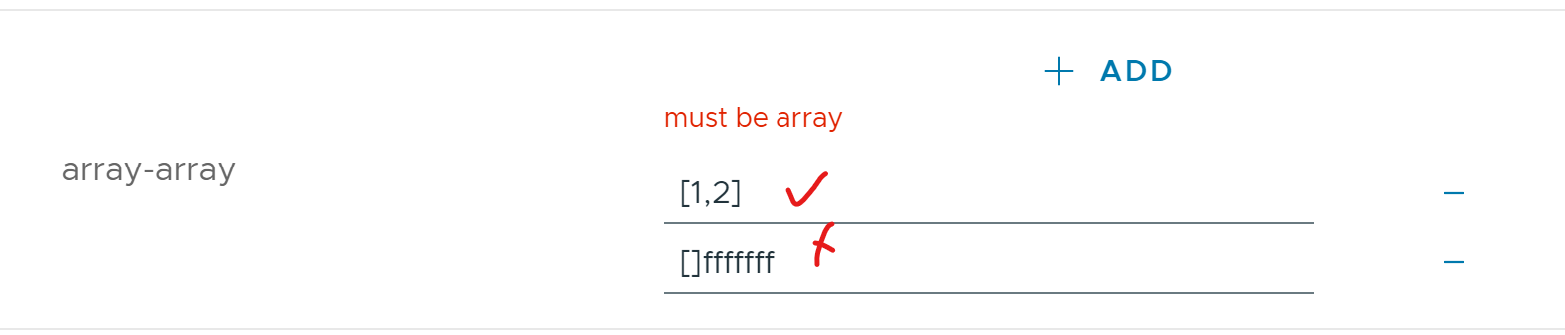 #### `Array<object>`:  #### `YAML output` 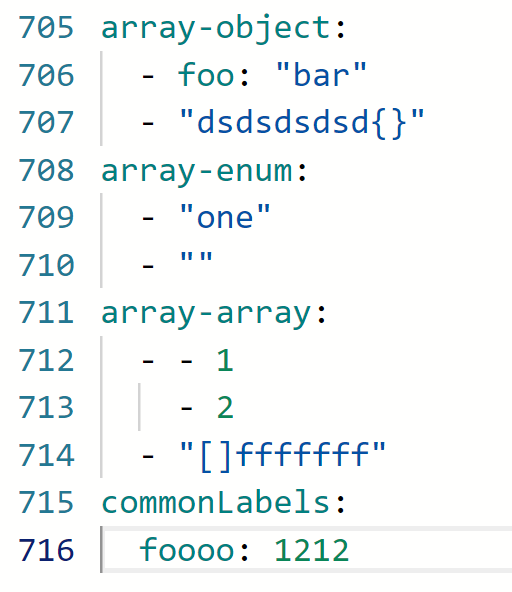 #### `Array max/min items` Min items:  Max items: 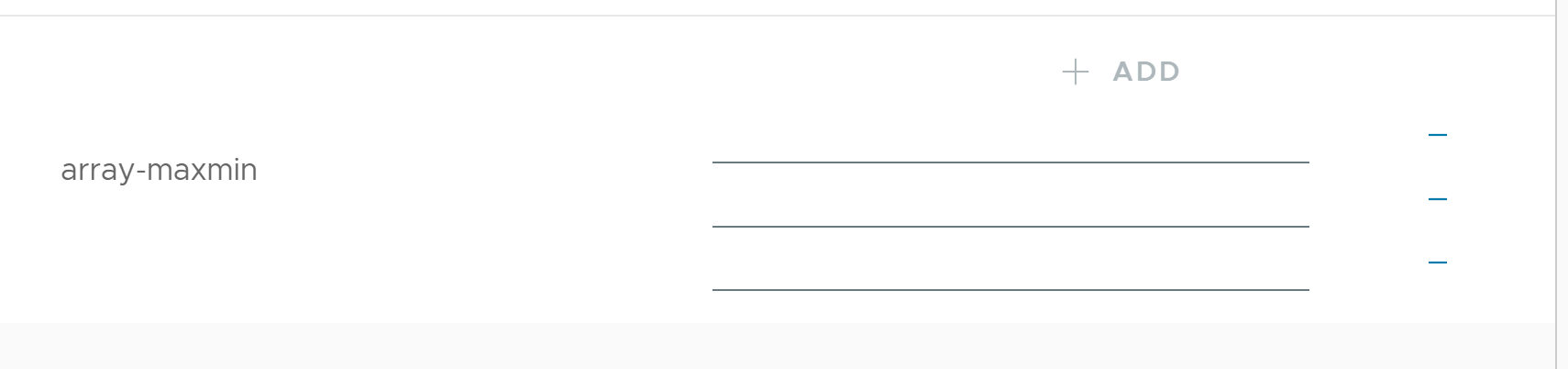 Signed-off-by: Antonio Gamez Diaz <[email protected]>
- Loading branch information
Showing
5 changed files
with
319 additions
and
188 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.